6.810 Engineering Interactive Technologies (fall 2021)
Pset1: User Interface for Multi-Touch Pad Fabrication Files
In this problem set, you will create a user interface that will automatically generate the fabrication files for making multi-touch pads of different dimensions (e.g., 8x4 electrodes or 4x6 electrodes). A user will be able to input parameters, such as the number of electrodes for the rows and columns of the multi-touch pad and then automatically receive the matching fabrication files, i.e. 2D drawings of the electrode layouts in .pdf file format that the user can then send to their 2D printer for conductive inkjet printing. You will implement this interface in Processing.For the multi-touch pad, we will use the classical two-layered diamond pattern that is commonly used for mutual-capacitive touch sensing. As explained in lecture, this means that your multi-touch pad has two electrode layers, printed separately: One layer contains the rows, the other contains the columns. Each row/column consists of multiple electrodes and has a single wire at the end. Once both sheets are printed, you will layer them on top of each other to build the multi-touch pad.
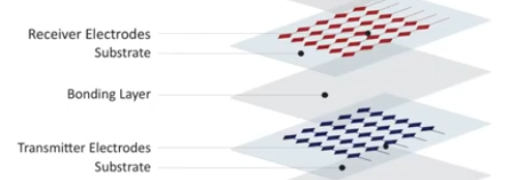
Skeleton Code:
Start by downloading the skeleton code for the PSet1 here.Steps:
- Create input fields for the number of electrodes
- Draw the electrodes and wires
- Create a preview function for looking at the rows/columns individually
- Create an export function to save the fabrication files as a .pdf
- Check that your drawing has the correct size when printed out
- Adjust the Multi-Touch Pad dimensions using DPI scale factor
- Export the Multi-touch Pad
- Check Multi-Touch Dimensions in mm in Illustrator
Help us Improve Class Materials for PSet1:
Please let us know if you found any bugs in the skeleton code or if anything was confusing in the write up.
You can add your comments here.
Please let us know if you found any bugs in the skeleton code or if anything was confusing in the write up.
You can add your comments here.
(1) Create Input Fields for the Number of Electrodes
In the user interface, create two input fields that allow the user to set the desired number of electrodes for the 'rows' and 'columns' (default value should be 10 electrodes for both). A simple trick to creating UI elements in Processing is to first draw a rectangle; then, you can check if a mouse-click event occured over the rectangle to create a button. You can also overlay a text label on the rectangle to display the current value. Refer to the Rect and Text and Fonts tutorials for more information. Make sure the user cannot enter negative values. The upper bound for the number of electrodes that fit on one sheet is 12 electrodes and thus the user should not be allowed to input values that exceed this number.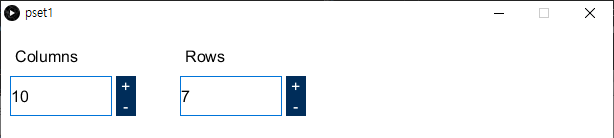
In the skeleton code, put your code for creating the input fields in the
createUI()
function:
(2) Drawing the Electrodes and Wires
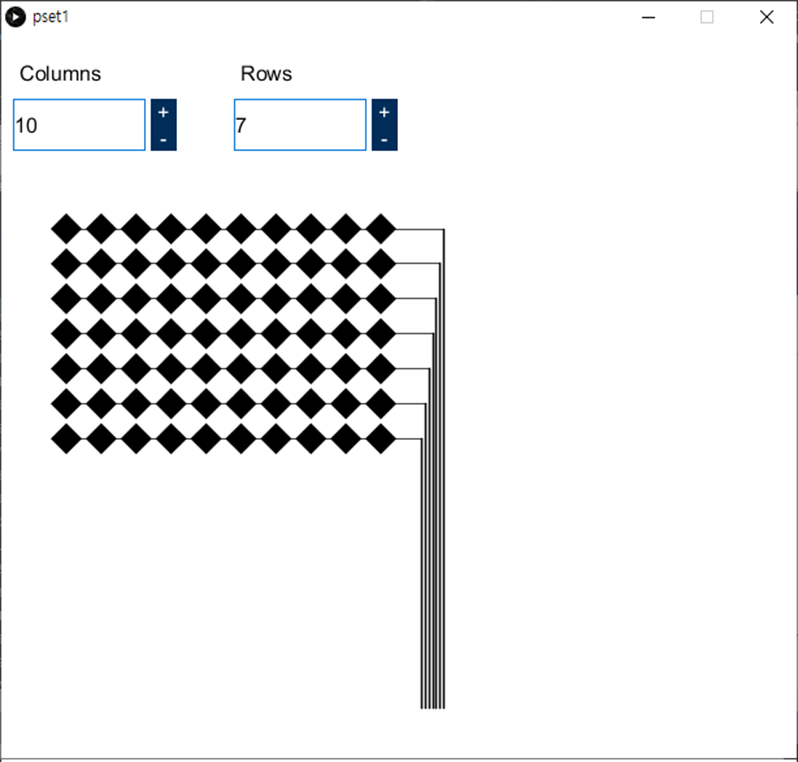
Drawing one electrode: Next, draw one electrode. An electrode is a rectangle rotated by 45 degrees. Use the variable
electrodeSize
from the pset1_header.pde file for the size of the electrode. Remember to draw the electrode in full black
so upon printing the inkjet printer will use the highest amount of silver ink for the area.In the skeleton code, put your code for drawing one electrode in the
drawSingleElectrode()
function:
Drawing multiple electrodes: Once you can draw one electrode, create a second electrode at an offset either horizontally to start forming a row, or vertically to start forming a column. Use the variable
electrodeOffset
from the pset1_header.pde file for the offset.
In the skeleton code, put your code for drawing multiple electrodes in the
drawElectrodes()
function:
Translate UI input into electrode count: Next, translate the UI input into the correct number of eletrodes generated by your functions, i.e. when the user sets the number of electrodes for the columns to be '10' and the rows to be '6', your program should draw a grid with 10 electrodes vertically and 6 electrodes horizontally.Drawing Wires: Next, draw the wires that connect the electrodes. Use the variables
wireThicknessInside
and wireThicknessOutside
from the pset1_header.pde file for the thickness of the wires.At the end of each row/column, the wire is routed to the side to connect to an FPC connector. Use the variable
spacing
in the pset1_header.pde file for the spacing on the FPC connector.
In the skeleton code, put your code for drawing wires in the
drawWires()
function:
(3) Preview Function to Look at Each Layer Individually
Top and Bottom Layer of Multi-touch Pad: The multi-touch pad consists of two layers; a bottom layer and a top layer. If the bottom layer has x rows and y columns of electrodes, the top layer should have y rows and x columns of electrodes. Thus, all you need to do to generate the second layer is to swap the row and column variables that the user entered.Create 'Switch Views' Button: Create a button that switches between the design's top and bottom layer view. That way, clicking the button should either show the top layer or the bottom layer so the layers can be looked at individually.
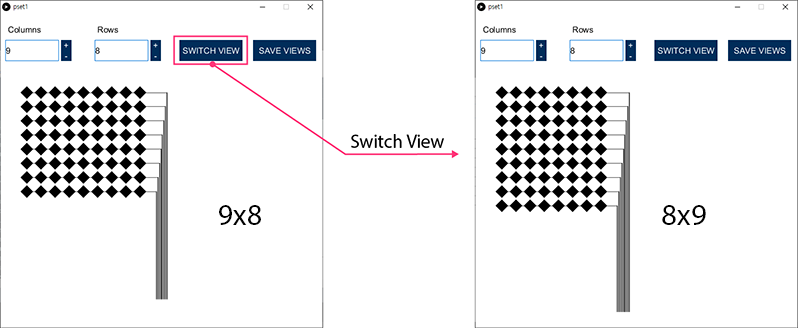
In the skeleton code, put your code for switching views between the design's top and bottom layer in the
switchView()
function:
(4) Export Function to Save Fabrication Files
Create 'Save Views' Button: Add a button that, when clicked, saves the generated electrode layers into two .pdf files. The bottom-electrodes.pdf should contain the rows, and the top-electrodes.pdf should contain the columns.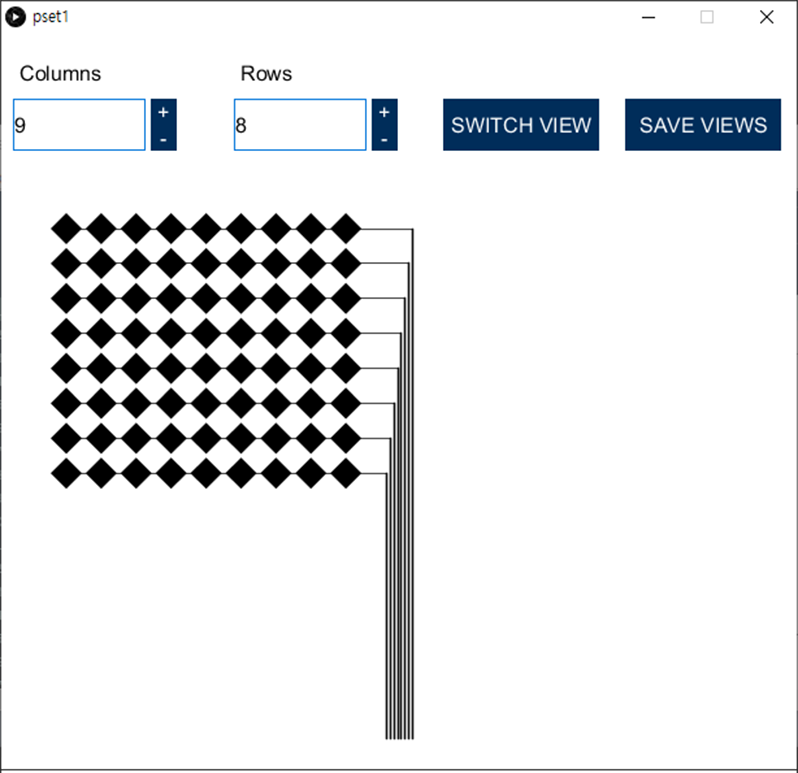
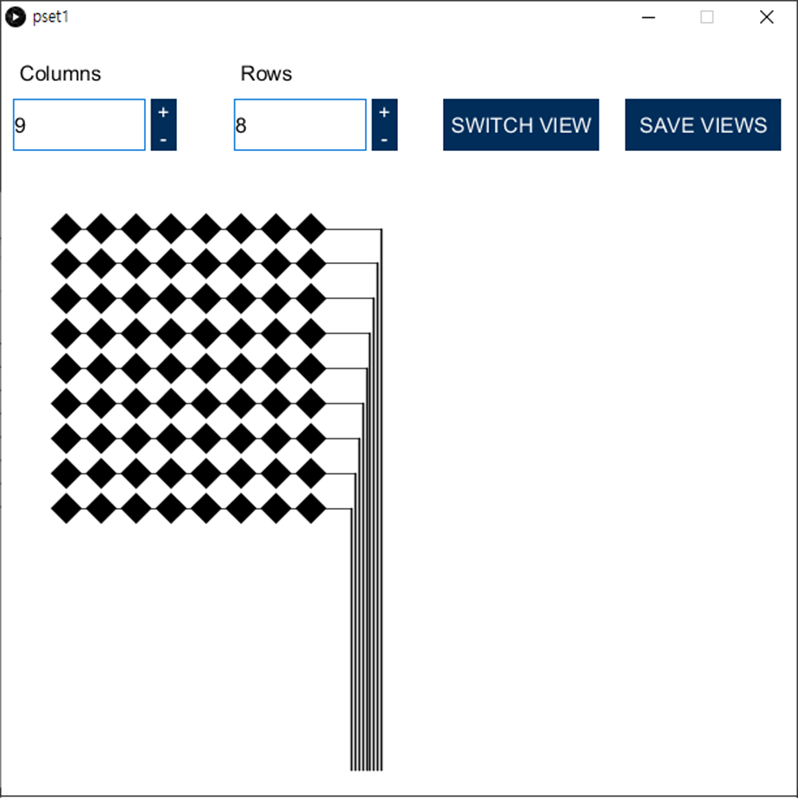
In the skeleton code, put your code for saving fabrication files in the
saveViews()
function:
(5) Finding Your DPI to Convert Pixel to mm
Our multi-touch pad design is printed in millimeters but Processing handles everything in pixels. How do we know the number of pixels in one millimeter? Without this conversion factor, we won't know how many pixels we should use for the electrodes lengths, wire thicknesses, and the spacing/offsets between these components. The conversion factor from pixel to mm is different for each operating system. We will thus do a test to figure this out for your specific OS.Determing DPI: Create a new document in Illustrator. Set the drawing unit to 'millimeters' (File -> Document Setup -> Units 'Milimeters').
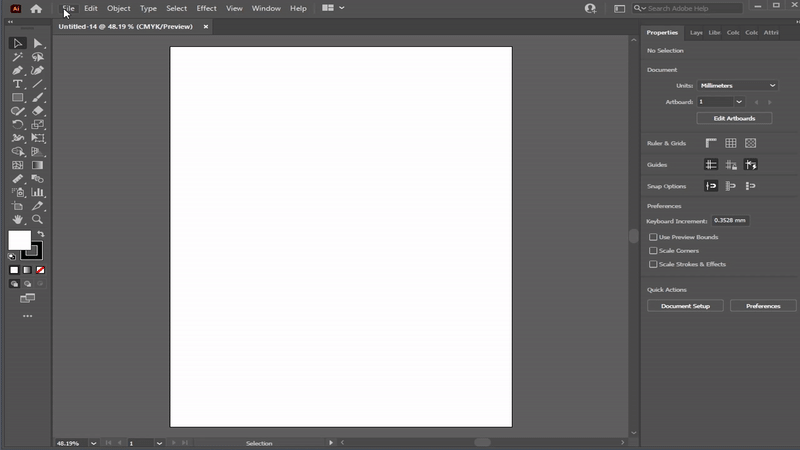
Then draw an 1 mm line with the pen tool. You can set line width to 1 mm and height to 0 mm in the property window.
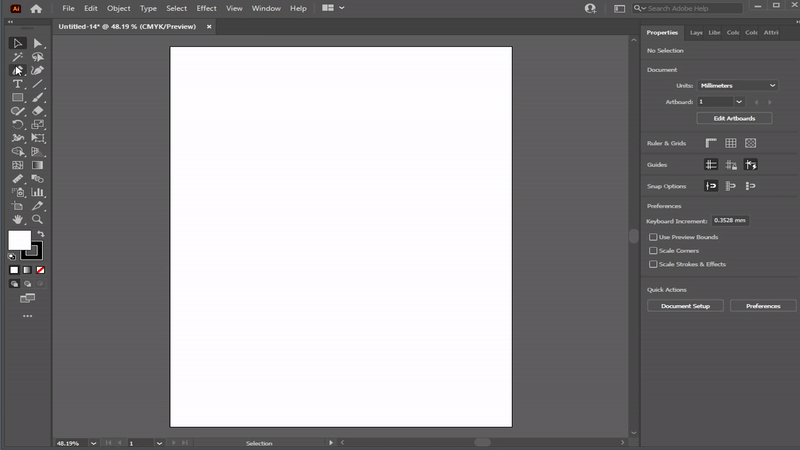
Change the drawing unit to 'pixels' (File -> Document Setup -> Units 'Pixels'). Then select the line to see the line length in the property panel in pixels.
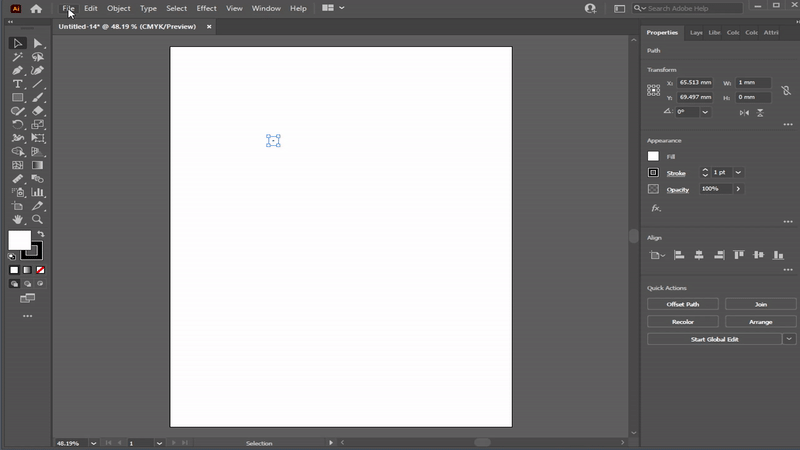
Now you know that 1 mm is a certain number of pixels (i.e. in our example 1mm is the same as 2.835 pixels but that value may be different for you because you have a different operating system).
Confirming dpi: To confirm that this is indeed the correct DPI for your OS, go back to Processing, and use the code below to draw a line of the number of pixels that Illustrator had showed you for 1mm (i.e in our case we draw a line of 2.835 pixels).
Export the line using your implemented .pdf export function, and open it in Adobe Illustrator. Don't forget to set the document units to 'milimeters'. Then select the line. Do you now see 1 mm? If yes, you can now be confident that 1mm is the number of pixels you thought (i.e. 2.835 pixels in our example).
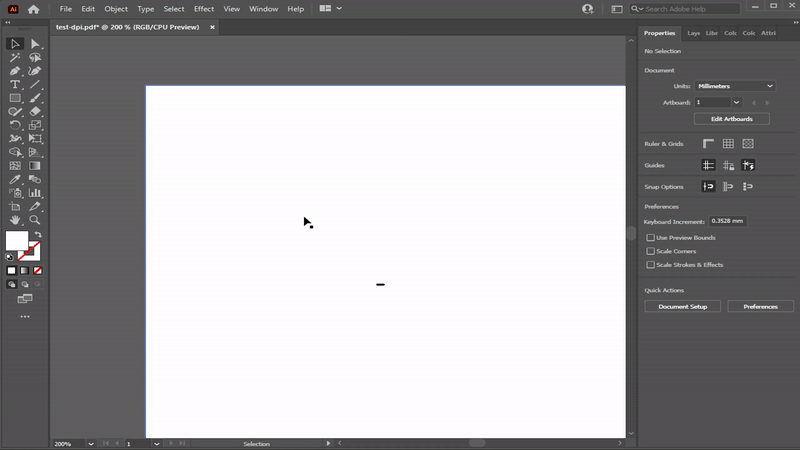
(6) Adjust Multi-Touch Pad Dimensions Using DPI Scale Factor
Now that you have your DPI scale factor, it's time to update all the multi-touch elements to have the right size.Applying Scaling Factor to Electrodes: To apply the scaling factor to everything you draw, we have created the variable
mm
in the skeleton code pset1_header.pde. Replace the value for mm
with the number of pixels you just determined in the previous section. Once you did this, all your drawn elements will be scaled appropriately.
So, if our electrodes are 6mm in size, they will be around 6 * 2.835 = 17.01 pixels wide.(7) Export Multi-touch Pad
When you are done with your code, export a multi-touch pad design of8x9 electrodes
from your user interface.Two PDF Files: You should have 2 pdf files: bottom-electrodes.pdf and top-electrodes.pdf.
(8) Check Multi-Touch Dimensions in mm in Illustrator
Now that you have the .pdf files, let's check in Illustrator that everything has the right size in mm and that both layers are aligned when placed on top of each other.How to Measure in Illustrator: Below is a short video of how to measure an element in Illustrator. Once you understood how to measure, read on to the next section to see what values you should expect to see for each part of your drawing.
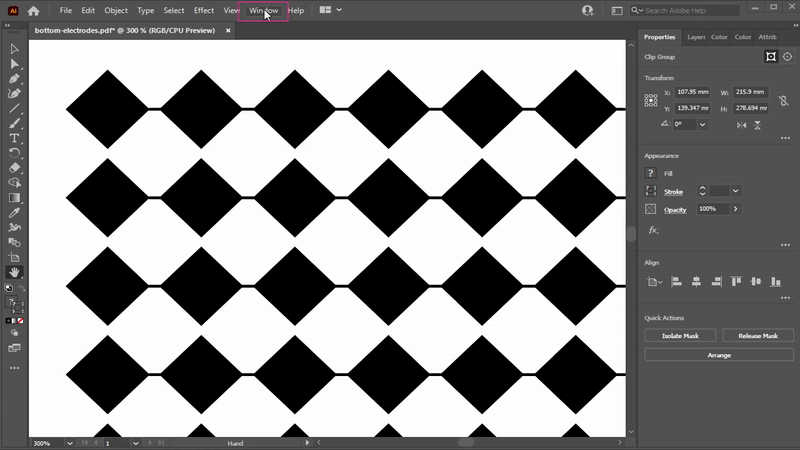
Check Dimensions in mm: Measure the length of the following elements to confirm that they have the right size (check the image/animation below for reference as well).
- edge length of the electrodes: 6mm
- spacing between connected electrodes: 1mm
- spacing between non-connected electrodes: 1mm
- thickness of wires inside of multi-touch pad that connect electrodes: 0.3mm
- thickness of the wires outside of multi-touch pad that connect to FPC connectors: 0.5mm
- spacing of wires on the FPC connector: 0.5mm
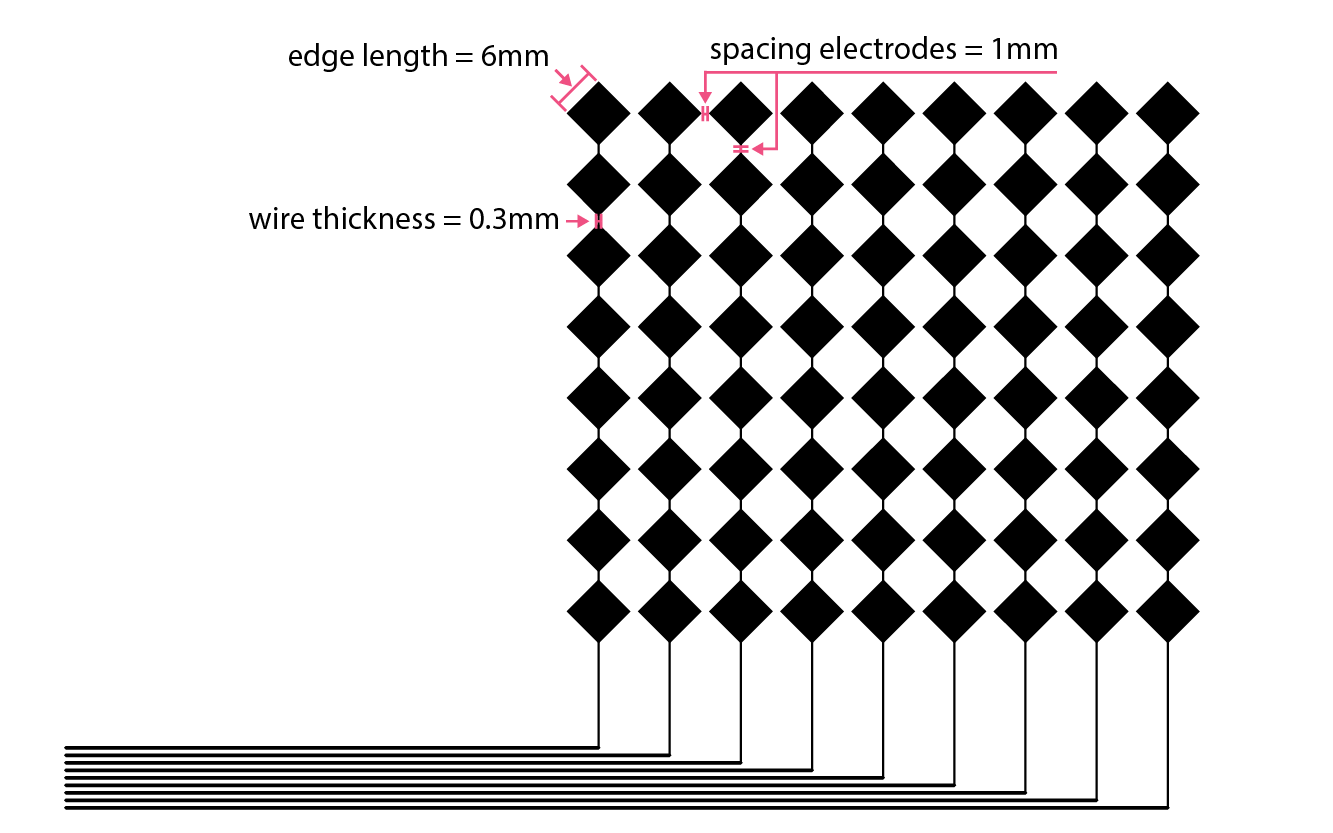
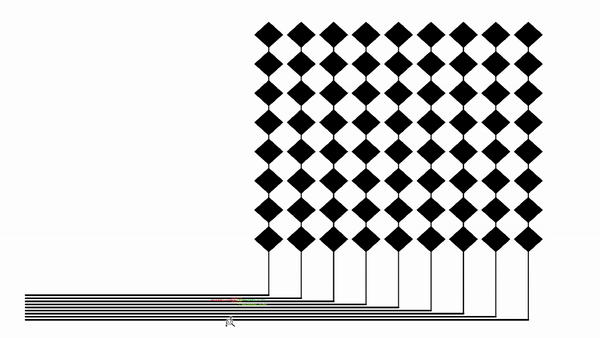
Check Alignment of both Top/Bottom Layers: Open both PDF files in Illustrator and overlay them on top of each other to check that the columns and rows of the multi-touch pad are correctly positioned.
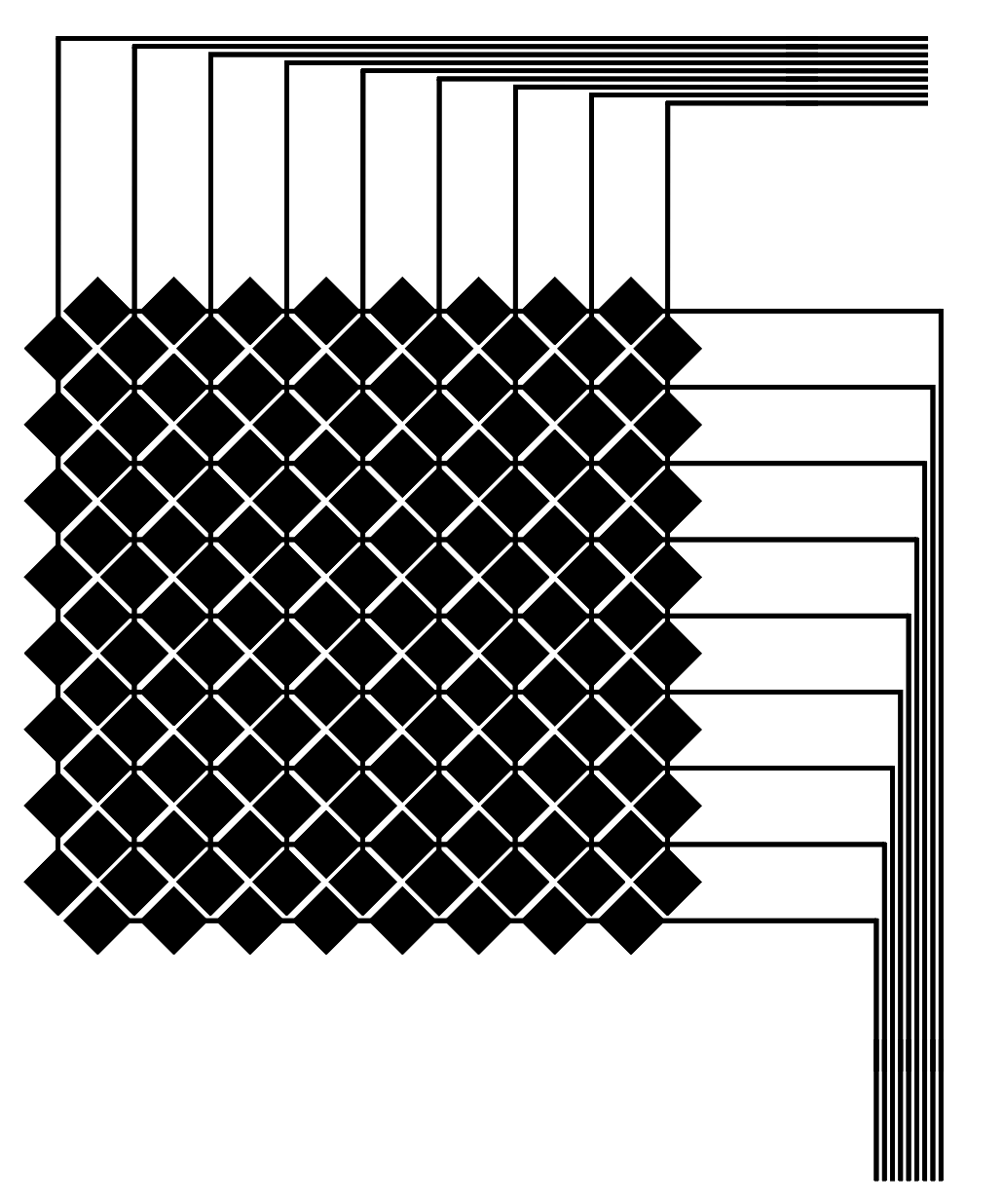
Deliverables
For grading, please upload the following to your google drive student folder:- the .pde files of your Processing program
- the two .pdf files for a multi-touch pad design of 8x9 electrodes, exported from your Processing program
Grading
We will give 20 pts in total:- 5pts: Does the Processing UI have input elements that set the number of electrodes in the x-direction and the number of electrodes in the y-direction
- 5pts: Is the layout of electrodes and wires correct? Are there wires going from the multi-touch pad to the FPC connector?
- 5pts: Does the Processing UI preview function show the top and bottom layers? Does the export function export the electrode layouts into two seperate .pdf files?
- 5pts: Was the dpi scale factor correctly determined, i.e. do the electrodes and wires have the correct length and spacing in the .pdf file?